Arduino Stroboscope Animation
UPDATE: Magzor has just started a Kickstarter campaign. Please check it out to get a good package deal on many of their components. Many of which were used in this tutorial.
This tutorial will show you how to build your own Stroboscopic Animator using Magzor's Mechanotronic Design Portal as a starting point. Magzor Corporation is a business in California that is trying really hard to simplify robotic design. They want to enable users with little to no engineering experience to design and manufacture a custom robot by themselves in a matter of hours.
What is a stroboscope? A stroboscope is an instrument that uses a strobe light to make a moving object look stationary… We will use this feature to create an interesting 4 picture animation on a rotating disk.
Have a look at the video below to see the project in action, and the MDP process walk-through:
Video
Parts Required:
- Arduino MEGA (or compatible board)
- Magzor I2C Arduino Shield
- Magzor MIC: Power and I2C board
- Magzor MIC: 10x GPIO
- Magzor MIC: 2x DC Motor Driver
- Magzor Hall Effect Breakout
- Neodymium Magnet
- Magzor LED board
- 6V 2.5A DC Planetary Geared Motor
- 5V DC 4A Power supply
- RJ45 cable
- Female to Female Jumper wire
- Misc items: scrap paper, wood, brackets, screws, single and double-sided tape, cardboard.
Magzor Schematic Diagram
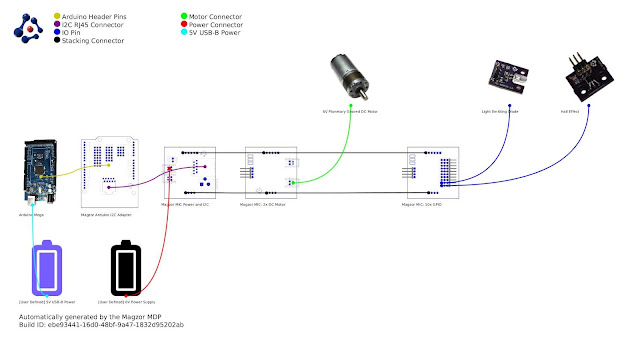
Further build instructions can be obtained by selecting the components in the Mechanotronics Design Portal within the Magzor website. Generating the build, and then selecting "Setup Instructions" tab at the top of the page. See video above to see this process in action.
Arduino Sketch
Make sure to copy and paste the following code into your Arduino IDE. It doesn't seem to work directly from the browser. You also need to install the Arduino Magzor I2C library ( http://magzor.com/downloads/ )
Putting it together

If you watch the video above, you will see that the image bounces around a little bit. The duration of each frame is determined by the speed of the rotating disk (or motor), and the number of LED flashes per frame. Any changes in rotation speed will affect the position of the picture when the LED blinks. My rotating disk is not completely semetrical or centred correctly, and therefore a bit jumpy… but you get the idea. Bold images with high contrast seem to work best… Precision is key for this type of project. And if you can get the disk to rotate at a constant speed, you could probably do away with the hall effect sensors and magnets… however, in my case, these were essential in getting the project to work as intended.
This project is a lot of fun. You can really get creative by making your own pictures or 3 dimensional models (for a stop motion effect). Try different colours. It really is quite cool.

Concluding Comments
I would like to thank Magzor for supplying the components used in this tutorial, and letting me try out their MDP process. I really like the concept, the one stop shop which looks after you from beginning to end. Providing everything I needed to get the project off the ground. The point of this exercise was to go through the entire process of selecting the parts, build the project, and get it up and running. And I have done that in no time at all.
There is only one library to download and install, and the good thing is that you don't have to go hunting for it. The latest "correct" working version of the library is easy to find, right there on the Magzor website… Speaking of the Magzor website, please make sure to take a quick look around. It is quite impressive.
UPDATE: Magzor has just started a Kickstarter campaign. Please check it out to get a good package deal on many of their components. Many of which were used in this tutorial.

Visit my ArduinoBasics Google + page.
Follow me on Twitter by looking for ScottC @ArduinoBasics.
I can also be found on Pinterest and Instagram.
Have a look at my videos on my YouTube channel.
Please visit their site and check out the MDP.

Feel free to share this page with your friends in any way you see fit.