The Need For Speed?
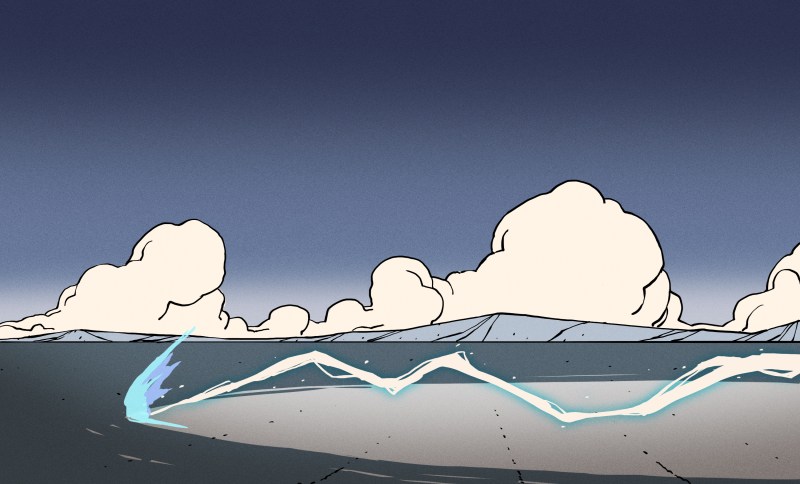
We wrote up a video about speeding up Arduino code, specifically by avoiding DigitalWrite
. Now, the fact that DigitalWrite
is slow as dirt is long known. Indeed, a quick search pulls up a Hackaday article from 2010 demonstrating that it’s fifty times slower than toggling the pin directly using the native pin registers, but this is still one of those facts that gets periodically rediscovered from generation to generation. How can this be new again?
First off, sometimes you just don’t need the speed. When you’re just blinking LEDs on a human timescale, the general-purpose Arduino functions are good enough. I’ve written loads of useful firmware that fits this description. When the timing requirements aren’t tight, slow as dirt can be fast enough.
But eventually you’ll want to build a project where the old slow-speed pin toggling just won’t cut it. Maybe it’s a large LED matrix, or maybe it’s a motor-control application where the loop time really matters. Or maybe it’s driving something like audio or video that just needs more bits per second. One way out is clever coding, maybe falling back to assembly language primitives, but I would claim that the right way is almost always to use the hardware peripherals that the chipmakers gave you.
For instance, in the end of the video linked above, the hacker wants to drive a large shift register string that’s lighting up an LED matrix. That’s exactly what SPI is for, and coming to this realization makes the project work with timing to spare, and in just a few lines of code. That is the way.
Which brings me to the double-edged sword that the Arduino’s abstraction creates. By abstracting away the chips’ hardware peripherals, it makes code more portable and certainly more accessible to beginners, who don’t want to learn about SPI and I2C and I2S and DMA just yet. But by hiding the inner workings of the chips in “user friendly” libraries, it blinds new users to the useful applications of these same hardware peripherals that clever chip-design engineers have poured their sweat and brains into making do just exactly what we need.
This isn’t really meant to be a rant against Arduino, though. Everyone has to start somewhere, and the abstractions are great for getting your feet wet. And because everything’s open source anyway, nothing stops you from digging deeper into the datasheet. You just have to know that you need to. And that’s why we write up videos like this every five years or so, to show the next crop of new hackers that there’s a lot to gain underneath the abstractions.