Simple Arduino Serial Communication (Part 2)
In Stages 1 to 5 , we experimented with the Serial Monitor on the Arduino IDE to transmit data to the Arduino and receive data from the Arduino. We performed simple Serial communication of data in both directions.
We then moved from transmitting a String of characters to the more difficult task of transmitting a double or a float.
Finally, we used the Serial monitor to receive sensor data from our Arduino, and played with a couple of Arduino functions.
We will now look at replacing the Serial Monitor with a much more exciting program such as "Processing" which is a free Open Source program that interfaces very easily with the Arduino. It can be downloaded here.
We will use a few processing scripts to bring our Arduino Sensor projects to life !
Stage 6: A simple Processing Sketch: BLINK
Arduino IDE vs Processing IDE
While the processing IDE looks very similar to the Arduino IDE, it is important that you realise that the Processing sketch will run on your computer, and NOT on the Arduino. In fact, you do not even need an Arduino to run a Processing sketch. And that is exactly what we are going to do: Run a Processing sketch without an Arduino.
Parts Required
Once you have downloaded and installed the Processing IDE onto your computer, open the program and copy the following sketch into it, and then press the play button. This is different from the Arduino IDE, in that we do not have to upload it to anything, as it will be running from the computer.
Processing Sketch
|
|
Things to Try
- Change the White background to a black background
- Increase the blink rate to 1 second
Insert on line 9: color Black=color(0,0,0);
Change line 38: background(Black);
Change line 25: delay(1000);
WARNING : Do not increase the speed too much, it may cause an epileptic fit.

Now that wasn't too hard I hope.
And if you want a very good site to learn Processing have a look at these
And if you want a very good site to learn Processing have a look at these

Stage 7: Arduino and Processing Unite.
So how do we get our Arduino to interface with our computer? Well, if the Serial Monitor on the Arduino IDE is not good enough, then you could use any program that is capable of Serial communication. Fortunately, Processing is one of those programs, but you could use C++, Java, Python, Microsoft Excel (using VBA), VB.Net, Gobetwino or some other programming language.
Two programs will be created: One will be uploaded to the Arduino using the Arduino IDE, and the other will run on the computer. In this example we will use Processing as the program that will run on the computer.
Two programs will be created: One will be uploaded to the Arduino using the Arduino IDE, and the other will run on the computer. In this example we will use Processing as the program that will run on the computer.
Enter the following sketch into the Arduino IDE and upload it to the Arduino. It serves to generate a random number between 0 and 400 every 200msec and will send it to the computer via the USB cable , using Serial communication.
Arduino Sketch
1 | /* Stage 7: Simple Arduino Serial Random Number Generator |
Instructions
Once the program has been uploaded to the Arduino, we will want to make sure that it is performing to expectations before moving onto the Processing script.
Open the Arduino Serial Monitor and make sure that you see a bunch of random numbers scrolling down the page. If not, then go back over your code and try again. Once you see the random numbers, then it is safe to move on to the next step.

Processing
The following Processing script will display the Random numbers being sent from the Arduino in the Processing IDE debug window. This particular script is very much like a simplified Arduino Serial Monitor, and it will show you that the Processing Script is successfully communicating with the Arduino.
Processing Sketch
1 | /* Some of the Serial code was adapted from Tom Igoe's example |
When you run the Processing script, a little black window will appear. This is the Processing Graphics window, which is normally where all the action takes place. However in the sketch above, this window does nothing. Instead we make use of the black Debug window which is part of the Processing IDE (below the code window). If everything went to plan, you should see random numbers scrolling down in a similar fashion to the Arduino Serial monitor. Here is an example of what it should look like.
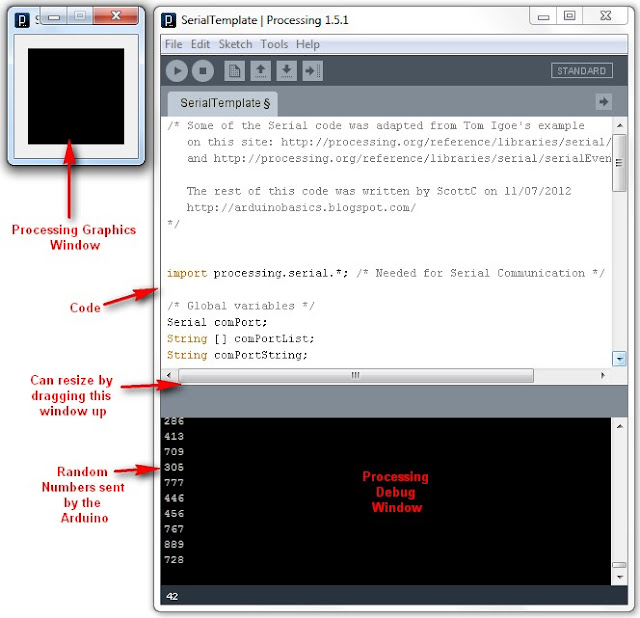

Things to Try
- If you are having problems with COM port selection. Then have a look at the COM port being used on the Arduino IDE to upload sketches to the Arduino. Processing generally uses the same COM port. So make sure to close the Arduino Serial Monitor before running the Processing Sketches.
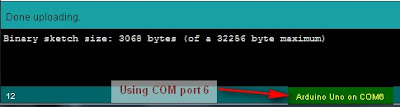
The image above shows that I am currently using COM PORT 6 on my computer to upload Arduino Sketches. In the Processing sketch on line 32-35, I had this code:
32 | if(comPortList.length>0){ |
We can change line 33 to get Processing to use COM port 6 exclusively. We do this by replacing comPortList[0] with "COM6", as seen below:
32 | if(comPortList.length>0){ |

Stage 8 : Arduino and Processing - Random Font Project
Arduino and Processing are speaking to each other by this stage, and we will keep our original Arduino Sketch to produce random numbers for us. Sure, we don't actually need the Arduino to do this for us, because Processing is more than capable of doing this itself, but we are building up towards an interactive sketch, so lets just go through the motions.
Just in case your mouse scroll wheel doesn't move upwards, here is the Arduino Sketch again:
Arduino Sketch
1 | /* Stage 8: Simple Arduino Serial Random Number Generator |
Upload the Arduino sketch to the Arduino, and then put the following code into the Processing IDE:
Processing Sketch

The output of this sketch should look something like this:
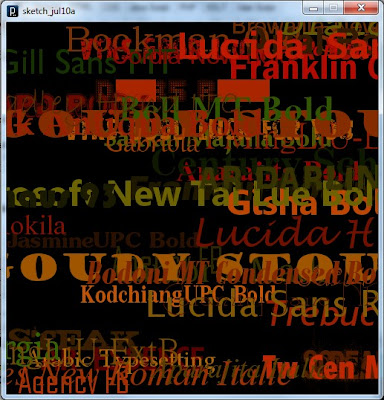

For stages 9 and above: Click Here
[original story: ScottC]